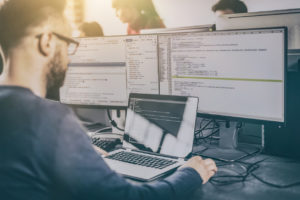
[From the last episode: We looked at several kinds of memories that are used with processorsA computer chip that does computing work for a computer. It may do general work (like in your home computer) or it may do specialized work (like some of the processors in your smartphone)..]
Before we go on to our next memory topic, we need to step aside for a second to explain a fundamental programming concept for those of you that may never have done any programming. You can likely skip this if you’re already a code jockey; it’s basic stuff for those of you that aren’t (and there’s no shame in that!).
We’re going to look at an important way both to reduce the amount of code in a program and to make the program easier to understand. That’s through the use of subroutinesA small portion of a program that’s set aside and given a name. It helps isolate key code in an easy-to-understand way, and it makes changes easier and less error prone. It’s a really important way to make programs easier to understand. May also be called a function. or functionsA small portion of a program that’s set aside and given a name. It helps isolate key code in an easy-to-understand way, and it makes changes easier and less error prone. It’s a really important way to make programs easier to understand. May also be called a subroutine.. (Exactly what they’re called may depend on the language you’re working with; I’ll use “subroutine” below.) We’ll use a ridiculously simple program to illustrate the notion.
Area Code
Let’s say that you have a program that needs to calculate the area of a bunch of circles. If the area of the circle is bigger than 100, then you want to do something – we’ll call the thing we’re going to do, GoDoIt. So you might have the following (fake) code:
r = 25 (assigns the value 25 to something called r) A = π*r^2 (where I use * to mean multiply and ^ to mean “to the power of”; so it’s pi-r-squared) if A > 100, then GoDoIt r = 5 A = π*r^2 if A > 100, then GoDoIt … etc.
First, we’ve got this thing called GoDoIt. We haven’t said what that does, but, in this part of the program, we don’t care. So we put those details away in a different part of the program, called a subroutine; we’ve named that subroutine GoDoIt. And you can see why that’s useful: we don’t have to worry about those details here. We worry about those details when we write that subroutine. In this part of the program, we simply call GoDoIt.
Let’s then focus just on the code above, ignoring the details of GoDoIt. What I’ve shown isn’t a huge bunch of code, so it might not look like a problem. But this is just to explain the concept. And, if we had a bunch more of the circles to evaluate, then our program might indeed look too long.
Simplify the Program
How do we simplify this and maybe organize it better? One thing we can look for is stuff we do over and over. For instance, we do the area calculation each time, only with a different number for the radius r. And we have to test every time. So what if we cut things down by putting those two things in their own subroutine and call it AreaOver100? AreaOver100 would take the radius as an input (not all subroutines take inputs, but most do), calculate the area, and test the result. AreaOver100 can also return a value (not all subroutines do): we can return TRUE or FALSE according to the test.
So the subroutine looks like this, using a fake-language definition to illustrate what’s happening:
Define subroutine AreaOver100 with input Radius and a True/False output Define a value Area for us to use only within this routine Area = π*Radius^2 If Area > 100, then AreaOver100 = TRUE Else AreaOver100 = FALSE End of AreaOver100
Now we can make the program a bit simpler:
if AreaOver100 (25) then GoDoIt (where the input to AreaOver100 is in the parentheses) if AreaOver100 (5) then GoDoIt etc. …
Each time we call AreaOver100, we pass a different number. The first time it’s 25. In the subroutine, Radius then gets the value 25 and the calculation happens. Next time it’s 5, and then Radius takes the value 5 and then does the calculation.
Easier to Understand and Maintain
You can see a couple of useful things that have happened here. First, we’ve simplified the original program; it’s much shorter and easier to read and understand. We’ve also taken a task that we need to do over and over and we’ve put it aside with its own name, AreaOver100. Now, if we ever want to make changes to how AreaOver100 works, we have to change it in only one place. If we used the original program, then we’d have to make those same changes over and over, everywhere we did the task.
Subroutines are simply a part of the program; this is about how we organize the program. When we compile the program into machine codeThe lowest-level representation of a computer program. It consists only of 1s and 0s, which a computer (or a machine) understands, but which is VERY hard for humans to understand., the subroutines are all in there, and the compilerA software development tool that takes a high-level program (source code) and translates it into a low-level machine-language program (object code). does the job of making sure the program knows where all the subroutines are so that it knows where to find them in memory when it needs them. (Exactly how this works depends on the language and is too fussy to worry about here.)
So – and this will be key next week – the instructions being executed within a program may bounce around as we go into and out of subroutines that might be all over the place in memory. We’re definitely not simply starting at the start and going in a straight line until the end. That’s an important thing to keep in mind.
You can check out the following mini-video to get a better idea of how this works.
(Yes, you’ll see in there a reference to a radius having a negative number. There are actually some contexts where that might make sense, but my goal was more to show that any number could work there — it doesn’t have to make physical sense. I haven’t invented some spooky hypercircle-thing. If this were a real program, that number probably wouldn’t be coming from the program itself, but from a user typing on a keyboard or something. Assuming we’re talking about real circles here, the program should then tell the user, “Wait, that makes no sense!” and wait until they entered a realistic number before executing CircleAreas. But that’s probably a discussion for a different time…)
Hi, Bryon, I think it would be useful to design and model a very basic computer that would actually run the subroutine/program.
I am working on a program that takes Boolean and arithmetic expressions as input and creates an executable model.
It has registers, flip-flops, and/or nets for control and will soon have arithmetic data flow for calculation.
Memory will be a simple integer array class that does load and store to an address in the array.
Visual Studio C# Community Edition is free and is great for debug/design entry as opposed to Verilog and VHDL.