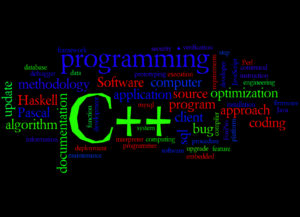
[From the last episode: We looked at the role of a processor in a typical systemThis is a very generic term for any collection of components that, all together, can do something. Systems can be built from subsystems. Examples are your cell phone; your computer; the radio in your car; anything that seems like a "whole.".]
We’ve taken a very brief, high-level look at a processor – and we’ll have lots more to say about them. But before we do that, let’s keep going with some of this big-picture stuff. As in, how the heck do you make a processor do the specific things you want? After all, that’s what a processor is good at – doing anything! (Even though it may not always be the best or fastest way to do it – it’s the most flexible.)
So what does a processor process? With apologies to any of you that may have been down this path already (not everyone has), it processes instructions. As in, you tell it what to do and, well, it does it. (Within reason.)
So… how do you tell it what to do? By writing a program. And how do you write that program? Well… it depends. Let’s start at the very lowest level.
Instruction Sets
Every processor has what is called an instruction setEach processor or processor family has a specific set of instructions that it can execute. This is called the instruction set, or sometimes the instruction-set architecture (ISA).. And each processor’s instruction set may be a little different. Some have very basic instructions, but they run really fast. Others have lots of complex instructions, but they may not be as speedy or they may use more energy. That whole balance between the speed you need, the energy you have access to (plugged into the wall? battery?), and the price is part of the decision as to which processor to use for a given job.
Those instructions have a specific format. Not only will the instructions vary by processor, but so will the machine-language format. We’ll make one up here just for illustration.
Let’s say that one of the instructions is to add two numbers, a and b. Usually it matters where a and b are stored, but we’re not going to worry about that. An instruction will consist of a code that means add and information on where to find a and b. All of that would typically go into a single instruction – in other words, you get both “what to do” and “what to do it to” in the one instruction.
Machine and Assembly Languages
All of that will be in 1s and 0s for the processor. That’s called machine languageThis is the lowest-level representation of a program – the 1s and 0s that the processor sees and understands. It’s very hard for humans to read., and it’s useful only to the processor. Humans can look at it, but it’s super hard to keep track of what’s going on if you have only machineIn our context, a machine is anything that isn't human (or living). That includes electronic equipment like computers and phones. language to look at. It might look something like,
(As a quick reminder, I’ve made up that code. Most real codes are far longer and more complicated.)
For humans, each processor has what’s called an assembly languageThis is a more human-readable version of machine language. It’s still low-level, but it uses text instead of 1s and 0s.. This is simply the bare instruction, but in human-readable form instead of 1s and 0s. Something like,
If you write a program in assembly language, then you use an assemblerA software development tool that takes a low-level assembly-language program (source code) and translates it into a machine-language program (object code). to turn it into machine language for the processor to run.
That’s much easier to understand than some cryptic bunch of 1s and 0s. But here’s the thing: each processor family will have its own assembly language; it’s not like there’s one assembly language for all processorsA computer chip that does computing work for a computer. It may do general work (like in your home computer) or it may do specialized work (like some of the processors in your smartphone).. You need machine language for the processor to know what you want, but you don’t want to write a program in machine language. Heck, you probably don’t want to write a program in assembly language either. You can do it, and some people do for really specific things where they want to get the best speed. But you have to take care of every little detail by hand, and it’s easy to make mistakes. So this isn’t great either.
High-Level Languages and Compilers
Now let’s move from the low level to a high level. Up here we have general programming languages. You might have heard of older ones like Fortran or Pascal or CA programming language that gives low-level control of all the details.. Then there are newer ones like C++ and Python and Perl and ones used for programming on the web. We want to program in these languages since they can do a whole lot more with fewer high-level instructions. And, unlike assembly language, a program we write in a high-level language may be usable on many different processors. How does that work?
It can work in one of two ways. The oldest method is by using a tool called a compilerA software development tool that takes a high-level program (source code) and translates it into a low-level machine-language program (object code).. A compiler translates a high-level human-understandable program into low-level assembly/machine code. Because those low-level formats are specific to different processors, it means you need a different compiler for each processor. If you buy a processor, you’re likely to get compilers and other tools from them as well. When you compile a program, you end up with a program that you can actually run on your processor. We call the high-level program source codeA program that’s readable by a human, usually in a high-level language. and the low-level compiled result object codeA program that’s been assembled, compiled, or translated into machine code.. (Don’t ask me why…)
With a compiler, you end up with a program that can simply run. But there’s a second way to do it: when it’s time to run the program, run the high-level program on some kind of interpreter that effectively does the same thing as a compiler, only it does it while the program is running, not ahead of time. You might run a high-level program as is, or you might translate the high-level program into some intermediate level that then gets interpreted as you run.
Of course, given a choice, compiling up front gives you the fastest program. Having to do the translation while it’s running takes time, so it’s a more flexible way to go, but it’s a bitThe smallest unit of information. It is a shortened form of "binary digit." Since it's binary, it can have only two values -- typically 0 and 1. slower.
We continue next with another high-level notion before digging into processors more.
Leave a Reply