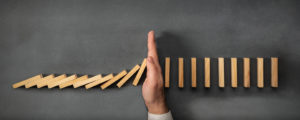
[From the last episode: We took a quick detour to look at the fates of several different IoTThe Internet of Things. A broad term covering many different applications where "things" are interconnected through the internet. servicesWe are used to purchasing products outright. "Services" is a new concept where you may or may not buy the product, but optional or mandatory services come with the product. Those services may have an ongoing cost separate from the purchase price..]
In our initial threadA piece of a program that can execute at the same time that some other piece of that same program can execute. discussion, we saw that a thread is, for lack of a better word, a task that a processorA computer chip that does computing work for a computer. It may do general work (like in your home computer) or it may do specialized work (like some of the processors in your smartphone). can do independently of – and even concurrently with – other tasks. That’s true, but it’s not quite as simple as just that. So this time, we’ll dig a bit more into what it means to be a thread.
Keeping it All in the Program
First, we need to make a big distinction. And we’ll do it in the context of a desktop computer to start with, since that’s familiar.
On your computer, you may run many different programs. You may have several of them open and active at the same time. That doesn’t make them threads.
Programmers have historically used the word process to describe what would typically be considered a program. It’s self-contained, relying only on itself and services provided by the operating systemSoftware – or firmware – that handles the low-level aspects of a computer or IoT device. It gets direct access to all the resources. Software asks the OS for access to those resources.. So having multiple programs open – say, Firefox and Thunderbird – means having multiple processes running.
Threads, by contrast, are part of a process. The process isn’t the thread; the thread is a piece of the process. If a program simply starts at the start and runs in one trajectory all the time, then that process has no threads. (Or you might say it has one thread, if you want…) Things get more interesting when a single process has multiple threads in play at the same time.
Of course, on your desktop, you may have multiple processes, each of which has multiple threads. So it’s entirely possible for there to be threads that don’t all belong to the same process. But whether or not they’re considered “threads” relates only to how their process (or program) is organized. We’ll use the following simple illustration to explain this.
Here we see three different programs, or processes. The main flow of the program is shown simply by a thicker black line. The orange pieces that look like they branch off are threads. The first program first has one thread that goes off, then another – and that one itself further spawns another thread. The second program shows no threads; the third one has one thread.
You Say Thread, I Say Tomahto
Now… why wouldn’t we consider the thick line also to be a thread, since it’s a flow of the program that’s running alongside the other threads. Ideally, you could consider it to be a thread. And, in some practical ways, it may act like a thread. But when programming, you start your program code and then, where needed, you ask the operating system to create a thread so that you can assign a task to it. In that way, a thread is something created within the main program. Program 2 above has no instructions that create a thread, which is why I show it as having no threads (as opposed to having one thread). It’s kind of a technicality, and, for our purposes, you can think of it either way.
There’s one other notion that the drawing illustrates. Most of the threads in the drawing go off and do something, and then they just end. They might be things like:
- Print a page.
- Display an error message with an “OK” button on it that the user will click.
- Take an email that’s ready to send and actually send it out onto the networkA collection of items like computers, printers, phones, and other electronic items that are connected together by switches and routers. A network allows the connected devices to talk to each other electronically. The internet is an example of an extremely large network. Your home network, if you have one, is an example of a small local network..
In all of these cases, there’s a task to do, you do the task, and then you’re done.
But you might notice that Program 1 has one thread that comes back to the main flow. Let’s make something up here: let’s say that the program needs to know how many files are on a hard driveA type of persistent (non-volatile) memory built from rotating platters and “read heads” that sense the data on the platters. for some reason*. So it creates a thread to go count the files; that’s shown as thread “a”. First it has to go see if the hard drive is attached; if it isn’t, then it creates thread “b” to give a message to the user and ends. Otherwise, it counts the files and returns to the main flow.**
I’m Depending on You
This returning thing has some implications if the main flow is counting on using the results that the thread brings back. If the main flow gets to that point and the thread hasn’t returned yet, then the main flow has to wait – it’s blocked. Such a thread is called a blockingIn the context of a thread, refers to execution that may have to wait for a result to come back before it can proceed. While waiting, it’s said to be blocked. thread. The others – the ones that just go off and then end – are non-blockingIn the context of a thread, refers to threads that can go off and do their thing without any other part of the program having to wait for them to finish. since nothing is waiting for them to finish.
The other notion that this illustrates is that of a dependency. The main flow needs to wait for the thread to come back, since what happens after depends on what number the thread returns with. DependenciesIn a computer program, refers to the situation where one piece of the program depends on the result of some other part of the program. If we calculate 1+1, then there’s no dependency. If we calculate a+b, then our result depends on whatever prior calculations produce a and b. are a big deal when it comes to pieces of programs that can run at the same time. We’ll be coming back to that notion later.
But… here’s an important question: how can two or more things run at the same time if there’s only one processor in the systemThis is a very generic term for any collection of components that, all together, can do something. Systems can be built from subsystems. Examples are your cell phone; your computer; the radio in your car; anything that seems like a "whole."?? We’ll talk about that next time.
* This isn’t how you would typically get a number of files; the operating system would most likely have that info. But it’s a simple example that’s easy to understand.
** Some of you that are more observant may wonder, if it couldn’t find the hard drive, puts up a message, and then ends, then what happens to the rest of the program, which is sitting there waiting for that message? In fact, the program would have to deal with this. But that adds more complication than I wanted, so I conveniently ignored it. If you read that and were wondering about that… then good for you! You’re thinking like a programmer!
Leave a Reply